mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 11:15:08 +00:00
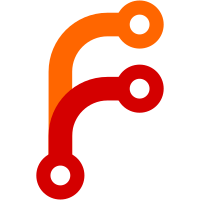
If a screen layout cannot be applied, instead of failing to start WindowServer try to fall back to an auto-generated screen layout with the devices that are detected. Also, be a bit smarter about changing the current screen layout. Instead of closing all framebuffers and bringing them back up, keep what we can and only change resolution on those that we need to change them on. To make this work we also need to move away from using an array of structures to hold compositor related per-screen data to attaching it to the Screen itself, which makes re-using a screen much simpler.
60 lines
1.5 KiB
C++
60 lines
1.5 KiB
C++
/*
|
|
* Copyright (c) 2020, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <AK/String.h>
|
|
#include <AK/Vector.h>
|
|
#include <LibCore/ConfigFile.h>
|
|
#include <LibGfx/Rect.h>
|
|
#include <LibGfx/Size.h>
|
|
#include <LibIPC/Forward.h>
|
|
|
|
namespace WindowServer {
|
|
|
|
class ScreenLayout {
|
|
public:
|
|
struct Screen {
|
|
String device;
|
|
Gfx::IntPoint location;
|
|
Gfx::IntSize resolution;
|
|
int scale_factor;
|
|
|
|
Gfx::IntRect virtual_rect() const
|
|
{
|
|
return { location, { resolution.width() / scale_factor, resolution.height() / scale_factor } };
|
|
}
|
|
|
|
bool operator==(const Screen&) const = default;
|
|
};
|
|
|
|
Vector<Screen> screens;
|
|
unsigned main_screen_index { 0 };
|
|
|
|
bool is_valid(String* error_msg = nullptr) const;
|
|
bool normalize();
|
|
bool load_config(const Core::ConfigFile& config_file, String* error_msg = nullptr);
|
|
bool save_config(Core::ConfigFile& config_file, bool sync = true) const;
|
|
bool try_auto_add_framebuffer(String const&);
|
|
|
|
// TODO: spaceship operator
|
|
bool operator!=(const ScreenLayout& other) const;
|
|
bool operator==(const ScreenLayout& other) const
|
|
{
|
|
return !(*this != other);
|
|
}
|
|
};
|
|
|
|
}
|
|
|
|
namespace IPC {
|
|
|
|
bool encode(Encoder&, const WindowServer::ScreenLayout::Screen&);
|
|
bool decode(Decoder&, WindowServer::ScreenLayout::Screen&);
|
|
bool encode(Encoder&, const WindowServer::ScreenLayout&);
|
|
bool decode(Decoder&, WindowServer::ScreenLayout&);
|
|
|
|
}
|