mirror of
https://github.com/RGBCube/serenity
synced 2025-07-19 00:47:35 +00:00
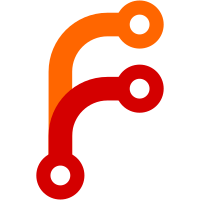
If there's no PCI bus, then it's safe to assume that the x86 machine we run on supports VGA text mode console output with an ISA VGA adapter. If this is the case, we just instantiate a ISAVGAAdapter object that assumes this situation and allows us to boot into VGA text mode console.
50 lines
1.1 KiB
C++
50 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2022, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <Kernel/Graphics/Console/ContiguousFramebufferConsole.h>
|
|
#include <Kernel/Graphics/Console/TextModeConsole.h>
|
|
#include <Kernel/Graphics/GraphicsManagement.h>
|
|
#include <Kernel/Graphics/VGA/ISAAdapter.h>
|
|
#include <Kernel/Sections.h>
|
|
|
|
namespace Kernel {
|
|
|
|
UNMAP_AFTER_INIT NonnullRefPtr<ISAVGAAdapter> ISAVGAAdapter::initialize()
|
|
{
|
|
return adopt_ref(*new ISAVGAAdapter());
|
|
}
|
|
|
|
UNMAP_AFTER_INIT ISAVGAAdapter::ISAVGAAdapter()
|
|
{
|
|
m_framebuffer_console = Graphics::TextModeConsole::initialize();
|
|
GraphicsManagement::the().set_console(*m_framebuffer_console);
|
|
}
|
|
|
|
void ISAVGAAdapter::enable_consoles()
|
|
{
|
|
VERIFY(m_framebuffer_console);
|
|
m_framebuffer_console->enable();
|
|
}
|
|
void ISAVGAAdapter::disable_consoles()
|
|
{
|
|
VERIFY(m_framebuffer_console);
|
|
m_framebuffer_console->disable();
|
|
}
|
|
|
|
void ISAVGAAdapter::initialize_framebuffer_devices()
|
|
{
|
|
}
|
|
|
|
bool ISAVGAAdapter::try_to_set_resolution(size_t, size_t, size_t)
|
|
{
|
|
return false;
|
|
}
|
|
bool ISAVGAAdapter::set_y_offset(size_t, size_t)
|
|
{
|
|
return false;
|
|
}
|
|
|
|
}
|