mirror of
https://github.com/RGBCube/serenity
synced 2025-07-14 21:37:37 +00:00
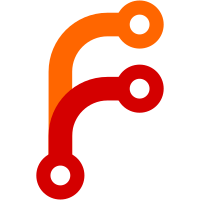
If the font dictionary didn't specify custom glyph widths, we would fall back to the specified "missing width" (or 0 in most cases!), which meant that we would draw glyphs on top of each other in a lot of cases, namely for TrueTypeFonts or standard Type1Fonts with an OpenType fallback. What we actually want to do in this case is ask the OpenType font for the correct width.
32 lines
1,015 B
C++
32 lines
1,015 B
C++
/*
|
|
* Copyright (c) 2023, Rodrigo Tobar <rtobarc@gmail.com>.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibGfx/Point.h>
|
|
#include <LibPDF/Fonts/PDFFont.h>
|
|
|
|
namespace PDF {
|
|
|
|
class SimpleFont : public PDFFont {
|
|
public:
|
|
PDFErrorOr<Gfx::FloatPoint> draw_string(Gfx::Painter&, Gfx::FloatPoint, DeprecatedString const&, Color const&, float font_size, float character_spacing, float horizontal_scaling) override;
|
|
|
|
protected:
|
|
PDFErrorOr<void> initialize(Document* document, NonnullRefPtr<DictObject> const& dict, float font_size) override;
|
|
virtual float get_glyph_width(u8 char_code) const = 0;
|
|
virtual void draw_glyph(Gfx::Painter& painter, Gfx::FloatPoint point, float width, u8 char_code, Color color) = 0;
|
|
RefPtr<Encoding>& encoding() { return m_encoding; }
|
|
RefPtr<Encoding> const& encoding() const { return m_encoding; }
|
|
|
|
private:
|
|
RefPtr<Encoding> m_encoding;
|
|
RefPtr<StreamObject> m_to_unicode;
|
|
HashMap<u8, u16> m_widths;
|
|
u16 m_missing_width;
|
|
};
|
|
|
|
}
|