mirror of
https://github.com/RGBCube/serenity
synced 2025-07-02 04:32:13 +00:00
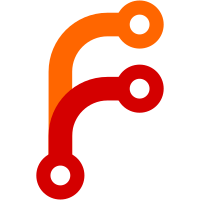
This is a variant of the enqueue() API that returns immediately and may fail. It's useful when you don't want to block until the audio server can receive your sample buffer.
43 lines
1.1 KiB
C++
43 lines
1.1 KiB
C++
#include <LibAudio/ABuffer.h>
|
|
#include <LibAudio/AClientConnection.h>
|
|
#include <SharedBuffer.h>
|
|
|
|
AClientConnection::AClientConnection()
|
|
: ConnectionNG("/tmp/asportal")
|
|
{
|
|
}
|
|
|
|
void AClientConnection::handshake()
|
|
{
|
|
auto response = send_sync<AudioServer::Greet>(getpid());
|
|
set_server_pid(response->server_pid());
|
|
set_my_client_id(response->client_id());
|
|
}
|
|
|
|
void AClientConnection::enqueue(const ABuffer& buffer)
|
|
{
|
|
for (;;) {
|
|
const_cast<ABuffer&>(buffer).shared_buffer().share_with(server_pid());
|
|
auto response = send_sync<AudioServer::EnqueueBuffer>(buffer.shared_buffer_id());
|
|
if (response->success())
|
|
break;
|
|
sleep(1);
|
|
}
|
|
}
|
|
|
|
bool AClientConnection::try_enqueue(const ABuffer& buffer)
|
|
{
|
|
const_cast<ABuffer&>(buffer).shared_buffer().share_with(server_pid());
|
|
auto response = send_sync<AudioServer::EnqueueBuffer>(buffer.shared_buffer_id());
|
|
return response->success();
|
|
}
|
|
|
|
int AClientConnection::get_main_mix_volume()
|
|
{
|
|
return send_sync<AudioServer::GetMainMixVolume>()->volume();
|
|
}
|
|
|
|
void AClientConnection::set_main_mix_volume(int volume)
|
|
{
|
|
send_sync<AudioServer::SetMainMixVolume>(volume);
|
|
}
|