mirror of
https://github.com/RGBCube/serenity
synced 2025-05-22 17:05:10 +00:00
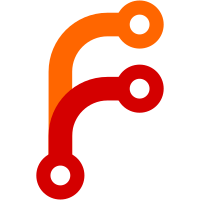
This is really a basic support for AHCI hotplug events, so we know how to add a node representing the device in /sys/dev/block and removing it according to the event type (insertion/removal). This change doesn't take into account what happens if the device was mounted or a read/write operation is being handled. For this to work correctly, StorageManagement now uses the Singleton container, as it might be accessed simultaneously from many CPUs for hotplug events. DiskPartition holds a WeakPtr instead of a RefPtr, to allow removal of a StorageDevice object from the heap. StorageDevices are now stored and being referenced to via an IntrusiveList to make it easier to remove them on hotplug event. In future changes, all of the stated above might change, but for now, this commit represents the least amount of changes to make everything to work correctly.
45 lines
1.1 KiB
C++
45 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2021, Liav A. <liavalb@hotmail.co.il>
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#include <AK/StringView.h>
|
|
#include <Kernel/FileSystem/OpenFileDescription.h>
|
|
#include <Kernel/Storage/AHCIController.h>
|
|
#include <Kernel/Storage/IDEChannel.h>
|
|
#include <Kernel/Storage/SATADiskDevice.h>
|
|
|
|
namespace Kernel {
|
|
|
|
NonnullRefPtr<SATADiskDevice> SATADiskDevice::create(const AHCIController& controller, const AHCIPort& port, size_t sector_size, u64 max_addressable_block)
|
|
{
|
|
return adopt_ref(*new SATADiskDevice(controller, port, sector_size, max_addressable_block));
|
|
}
|
|
|
|
SATADiskDevice::SATADiskDevice(const AHCIController& controller, const AHCIPort& port, size_t sector_size, u64 max_addressable_block)
|
|
: StorageDevice(controller, sector_size, max_addressable_block)
|
|
, m_port(port)
|
|
{
|
|
}
|
|
|
|
SATADiskDevice::~SATADiskDevice()
|
|
{
|
|
}
|
|
|
|
StringView SATADiskDevice::class_name() const
|
|
{
|
|
return "SATADiskDevice";
|
|
}
|
|
|
|
void SATADiskDevice::start_request(AsyncBlockDeviceRequest& request)
|
|
{
|
|
m_port.strong_ref()->start_request(request);
|
|
}
|
|
|
|
String SATADiskDevice::storage_name() const
|
|
{
|
|
return String::formatted("hd{:c}", 'a' + minor());
|
|
}
|
|
|
|
}
|