mirror of
https://github.com/RGBCube/serenity
synced 2025-07-16 01:57:39 +00:00
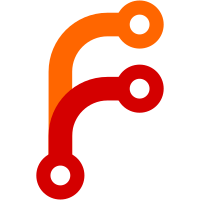
This change also adds non-deprecated text() and set_text() functions and helper constructors for Button, CheckBox, and RadioButton to call the strings directly. The whole codebase at this point is still using the deprecated string functions, which the class will quietly convert to a new String.
49 lines
1.1 KiB
C++
49 lines
1.1 KiB
C++
/*
|
|
* Copyright (c) 2018-2020, Andreas Kling <kling@serenityos.org>
|
|
* Copyright (c) 2022, the SerenityOS developers.
|
|
*
|
|
* SPDX-License-Identifier: BSD-2-Clause
|
|
*/
|
|
|
|
#pragma once
|
|
|
|
#include <LibGUI/AbstractButton.h>
|
|
|
|
namespace GUI {
|
|
|
|
class CheckBox : public AbstractButton {
|
|
C_OBJECT(CheckBox);
|
|
|
|
public:
|
|
virtual ~CheckBox() override = default;
|
|
|
|
virtual void click(unsigned modifiers = 0) override;
|
|
|
|
bool is_autosize() const { return m_autosize; }
|
|
void set_autosize(bool);
|
|
|
|
enum class CheckBoxPosition {
|
|
Left,
|
|
Right,
|
|
};
|
|
CheckBoxPosition checkbox_position() const { return m_checkbox_position; }
|
|
void set_checkbox_position(CheckBoxPosition value) { m_checkbox_position = value; }
|
|
|
|
protected:
|
|
explicit CheckBox(DeprecatedString);
|
|
explicit CheckBox(String = {});
|
|
|
|
private:
|
|
void size_to_fit();
|
|
|
|
// These don't make sense for a check box, so hide them.
|
|
using AbstractButton::auto_repeat_interval;
|
|
using AbstractButton::set_auto_repeat_interval;
|
|
|
|
virtual void paint_event(PaintEvent&) override;
|
|
|
|
bool m_autosize { false };
|
|
CheckBoxPosition m_checkbox_position { CheckBoxPosition::Left };
|
|
};
|
|
|
|
}
|