mirror of
https://github.com/RGBCube/serenity
synced 2025-05-25 15:05:07 +00:00
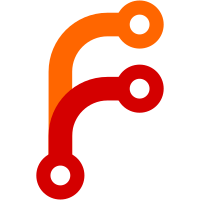
Walk the DOM and construct a parallel style tree that points back to the DOM and has the relevant CSS property values hanging off of them. The values are picked based on naive selector matching. There's no cascade or specificity taken into account yet.
53 lines
1.3 KiB
C++
53 lines
1.3 KiB
C++
#pragma once
|
|
|
|
#include <AK/HashMap.h>
|
|
#include <AK/NonnullRefPtr.h>
|
|
#include <AK/AKString.h>
|
|
#include <LibHTML/TreeNode.h>
|
|
#include <LibHTML/CSS/StyleValue.h>
|
|
|
|
class Node;
|
|
|
|
class StyledNode : public TreeNode<StyledNode> {
|
|
public:
|
|
static NonnullRefPtr<StyledNode> create(const Node& node)
|
|
{
|
|
return adopt(*new StyledNode(&node));
|
|
}
|
|
~StyledNode();
|
|
|
|
const Node* node() const { return m_node; }
|
|
|
|
template<typename Callback>
|
|
inline void for_each_child(Callback callback) const
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void for_each_child(Callback callback)
|
|
{
|
|
for (auto* node = first_child(); node; node = node->next_sibling())
|
|
callback(*node);
|
|
}
|
|
|
|
template<typename Callback>
|
|
inline void for_each_property(Callback callback) const
|
|
{
|
|
for (auto& it : m_property_values)
|
|
callback(it.key, *it.value);
|
|
}
|
|
|
|
void set_property(const String& name, NonnullRefPtr<StyleValue> value)
|
|
{
|
|
m_property_values.set(name, move(value));
|
|
}
|
|
|
|
protected:
|
|
explicit StyledNode(const Node*);
|
|
|
|
private:
|
|
const Node* m_node { nullptr };
|
|
HashMap<String, NonnullRefPtr<StyleValue>> m_property_values;
|
|
};
|